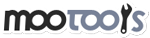
A collection of the String Object prototype methods.
Pads a string with the specified character(s) either before or after the current value.
myString.pad(length, string, direction);
var tonyTheTigerSez = "They're gr".pad(15, 'r') + 'eat!'; //returns "They're grrrrrreat!"
Repeats a string a specified number of times.
myString.repeat(times);
var one = "1"; var eleventyOne = one.repeat(3); //returns "111"
Removes non-ascii characters and converts them to their most appropriate ascii character.
myString.standardize();
var bjorkProper = "Björk"; var bjorkAscii = bjorkProper.standardize(); //returns "Bjork"
Get all the HTML tags from a given string.
myString.getTags([tag, contents]);
var html = "<b>This is a string with <i>html</i> in it.</b>" var tags = html.getTags(); //returns ["<b>", "<i>", "</i>", "</b>"] var italics = html.getTags('i'); //returns ["<i>", "</i>"] var italicsWithContent = html.getTags('i', true); //returns ["<i>html</i>"]
Currently, you cannot ask for all tags with their content. If you want the inner content of tags, you must specify the tag type.
Remove all html tags from a string.
myString.stripTags([tag, contents]);
var html = "<b>This is a string with <i>html</i> in it.</b>" var noHtml = html.stripTags(); //returns "This is a string with html in it." var noItalics = html.stripTags('i'); //returns "<b>This is a string with html in it.</b>" var noItalicsContent = html.stripTags('i', true); returns "<b>This is a string with in it.</b>"
Replaces common special characters with their ASCII counterparts (smart quotes, elipse characters, stuff from MS Word, etc.).
var tidyString = stringWithBadChars.tidy();
Truncates a string after the given number of characters.
myString.truncate(max, trail, atChar);
â¦
when only the max
argument is passed.'This is some random text'.truncate(15); // This is some ra⦠'This is some random text'.truncate(15, '--'); // This is some ra-- 'This is some random text'.truncate(15, 'â¦', ' '); // This is someâ¦
© Linux.ria.ua, 2008-2024 |