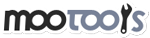
The base Class of the MooTools framework.
var MyClass = new Class(properties);
The methods of this Class that have the same name as the Extends Class, will have a parent property, that allows you to call the other overridden method. The Extends property should be the first property in a class definition.
Implements is similar to Extends, except that it adopts properties from one or more other classes without inheritance. Useful when implementing a default set of properties in multiple Classes. The Implements property should come after Extends but before all other properties.
var Cat = new Class({ initialize: function(name){ this.name = name; } }); var myCat = new Cat('Micia'); alert(myCat.name); // alerts 'Micia' var Cow = new Class({ initialize: function(){ alert('moooo'); } });
var Animal = new Class({ initialize: function(age){ this.age = age; } }); var Cat = new Class({ Extends: Animal, initialize: function(name, age){ this.parent(age); // calls initalize method of Animal class this.name = name; } }); var myCat = new Cat('Micia', 20); alert(myCat.name); // alerts 'Micia'. alert(myCat.age); // alerts 20.
var Animal = new Class({ initialize: function(age){ this.age = age; } }); var Cat = new Class({ Implements: Animal, setName: function(name){ this.name = name } }); var myAnimal = new Cat(20); myAnimal.setName('Micia'); alert(myAnimal.name); // alerts 'Micia'.
Implements the passed in properties into the base Class prototypes, altering the base Class. The same as creating a new Class with the Implements property, but handy when you need to modify existing classes.
MyClass.implement(properties);
var Animal = new Class({ initialize: function(age){ this.age = age; } }); Animal.implement({ setName: function(name){ this.name = name; } }); var myAnimal = new Animal(20); myAnimal.setName('Micia'); alert(myAnimal.name); // alerts 'Micia'
© Linux.ria.ua, 2008-2024 |