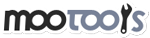
Allows for the animation of multiple CSS properties at once, even by a simple CSS selector. Inherits methods, properties, options and events from Fx.
var myFx = new Fx.Morph(element[, options]);
Multiple styles with start and end values using an object:
var myEffect = new Fx.Morph('myElement', { duration: 'long', transition: Fx.Transitions.Sine.easeOut }); myEffect.start({ 'height': [10, 100], // Morphs the 'height' style from 10px to 100px. 'width': [900, 300] // Morphs the 'width' style from 900px to 300px. });
Multiple styles with the start value omitted will default to the current Element's value:
var myEffect = new Fx.Morph('myElement', { duration: 'short', transition: Fx.Transitions.Sine.easeOut }); myEffect.start({ 'height': 100, // Morphs the height from the current to 100px. 'width': 300 // Morphs the width from the current to 300px. });
Morphing one Element to match the CSS values within a CSS class. This is useful when separating the logic and styles:
var myEffect = new Fx.Morph('myElement', { duration: 1000, transition: Fx.Transitions.Sine.easeOut }); // the styles of myClassName will be applied to the target Element. myEffect.start('.myClassName');
Sets the Element's CSS properties to the specified values immediately.
myFx.set(to);
var myFx = new Fx.Morph('myElement').set({ 'height': 200, 'width': 200, 'background-color': '#f00', 'opacity': 0 }); var myFx = new Fx.Morph('myElement').set('.myClass');
Executes a transition for any number of CSS properties in tandem.
myFx.start(properties);
var myEffects = new Fx.Morph('myElement', {duration: 1000, transition: Fx.Transitions.Sine.easeOut}); myEffects.start({ 'height': [10, 100], 'width': [900, 300], 'opacity': 0, 'background-color': '#00f' });
Sets a default Fx.Morph instance for an Element.
el.set('morph'[, options]);
el.set('morph', {duration: 'long', transition: 'bounce:out'}); el.morph({height: 100, width: 100});
Gets the default Fx.Morph instance for the Element.
el.get('morph');
el.set('morph', {duration: 'long', transition: 'bounce:out'}); el.morph({height: 100, width: 100}); el.get('morph'); // the Fx.Morph instance.
Animates an Element given the properties passed in.
myElement.morph(properties);
With an object:
$('myElement').morph({height: 100, width: 200});
With a selector:
$('myElement').morph('.class1');
© Linux.ria.ua, 2008-2024 |