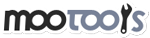
Extends the Element type to include event-delegation in its event system.
Event delegation is a common practice where an event listener is attached to a parent element to monitor its children rather than attach events to every single child element. It's more efficient for dynamic content or highly interactive pages with a lot of DOM elements.
An example of how delegation is usually applied. Delegation is extra useful when working with dynamic content like AJAX.
var myElement = $('myElement'); var request = new Request({ // other options onSuccess: function(text){ myElement.set('html', text); // No need to attach more click events. } }); // Adding the event, notice the :relay syntax with the selector that matches the target element inside of myElement. // Every click on an anchor-tag inside of myElement executes this function. myElement.addEvent('click:relay(a)', function(event, target){ event.preventDefault(); request.send({ url: target.get('href') }); });
Delegates the methods of an element's children to the parent element for greater efficiency when a selector is provided. Otherwise it will work like addEvent.
myElement.addEvent(typeSelector, fn);
// Alerts when an anchor element with class 'myStyle' is clicked inside of myElement document.id(myElement).addEvent('click:relay(a.myStyle)', function(){ alert('hello'); }); document.id('myElement').addEvent('click:relay(a)', function(event, clicked){ event.preventDefault(); //don't follow the link alert('you clicked a link!'); // You can reference the element clicked with the second // Argument passed to your callback clicked.setStyle('color', '#777'); });
Delegates the events to the parent just as with addEvent above. Works like addEvents.
$('myElement').addEvents({ // monitor an element for mouseover mouseover: fn, // but only monitor child links for clicks 'click:relay(a)': fn2 });
Removes a method from an element like removeEvent.
var monitor = function(event, element){ alert('you clicked a link!'); }; $('myElement').addEvent('click:relay(a)', monitor); // link clicks are delegated to element // remove delegation: $('myElement').removeEvent('click:relay(a)', monitor);
Removes a series of methods from delegation if the functions were used for delegation or else works like removeEvents.
var monitor = function(){ alert('you clicked or moused over a link!'); }; $('myElement').addEvents({ 'mouseover:relay(a)': monitor, 'click:relay(a)': monitor }); // link clicks are delegated to element // remove the delegation: $('myElement').removeEvents({ 'mouseover:relay(a)': monitor, 'click:relay(a)': monitor }); // remove all click:relay(a) events $('myElement').removeEvents('click:relay(a)');
© Linux.ria.ua, 2008-2024 |