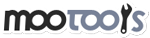
The following functions are treated as Window methods.
The document.id function has a dual purpose: Getting the element by its id, and making an element in Internet Explorer "grab" all the Element methods.
var myElement = document.id(el);
var myElement = document.id('myElement');
var div = document.getElementById('myElement'); div = document.id(div); // the element with all the Element methods applied.
The dollar function is an alias for document:id if the $ variable is not set already. However it is not recommended to use more frameworks, the $ variable can be set by another framework or script. MooTools will detect this and determine if it will set the $ function so it will not be overwritten.
var myElement = $('myElement'); var myElement2 = document.id('myElement'); myElement == myElement2; // returns true (function($){ // Now you can use $ safely in this closure })(document.id)
Selects and extends DOM elements. Return an Elements instance. The Element instance returned is an array-like object, supporting every Array method and every Element method.
var myElements = $$(argument);
$$('a'); // returns all anchor elements in the page.
$$(element1, element2, element3); // returns an Elements instance containing these 3 elements.
$$([element1, element2, element3]); // returns an Elements instance containing these 3 elements. $$(document.getElementsByTagName('a')); // returns an Elements instance containing the result of the getElementsByTagName call.
$$('#myElement'); // returns an Elements instance containing only the element with the id 'myElement'. $$('#myElement a.myClass'); // returns an Elements instance of all anchor tags with the class 'myClass' within the DOM element with id 'myElement'. $$('a, b'); // returns an array of all anchor and bold elements in the page.
Custom Type to allow all of its methods to be used with any extended DOM Element.
Creates a new Element of the type passed in.
var myEl = new Element(element[, properties]);
// Creating an new anchor with an Object var myAnchor = new Element('a', { href: 'http://mootools.net', 'class': 'myClass', html: 'Click me!', styles: { display: 'block', border: '1px solid black' }, events: { click: function(){ alert('clicked'); }, mouseover: function(){ alert('mouseovered'); } } }); // Using Selectors var myNewElement = new Element('a.myClass');
Because the element name is parsed as a CSS selector, colons in namespaced tags have to be escaped. So new Element('fb\:name)
becomes <fb:name>
.
Gets the first descendant element whose tag name matches the tag provided. CSS selectors may also be passed.
var myElement = myElement.getElement(tag);
var firstDiv = $(document.body).getElement('div');
Collects all decedent elements whose tag name matches the tag provided. CSS selectors may also be passed.
var myElements = myElement.getElements(tag);
var allAnchors = $(document.body).getElements('a');
Gets the element with the specified id found inside the current Element.
var myElement = anElement.getElementById(id);
var myChild = $('myParent').getElementById('myChild');
This is a "dynamic arguments" method. Properties passed in can be any of the 'set' properties in the Element.Properties Object.
myElement.set(arguments);
$('myElement').set('text', 'text goes here'); $('myElement').set('class', 'active'); // the 'styles' property passes the object to Element:setStyles. var body = $(document.body).set('styles', { font: '12px Arial', color: 'blue' });
var myElement = $('myElement').set({ // the 'styles' property passes the object to Element:setStyles. styles: { font: '12px Arial', color: 'blue', border: '1px solid #f00' }, // the 'events' property passes the object to Element:addEvents. events: { click: function(){ alert('click'); }, mouseover: function(){ this.addClass('over'); } }, //Any other property uses Element:setProperty. id: 'documentBody' });
type
multiple times. It will throw an error.This is a "dynamic arguments" method. Properties passed in can be any of the 'get' properties in the Element.Properties Object.
myElement.get(property);
var tag = $('myDiv').get('tag'); // returns "div".
var id = $('myDiv').get('id'); // returns "myDiv". var value = $('myInput').get('value'); // returns the myInput element's value.
This is a "dynamic arguments" method. Properties passed in can be any of the 'erase' properties in the Element.Properties Object.
myElement.erase(property);
$('myDiv').erase('id'); //Removes the id from myDiv. $('myDiv').erase('class'); //myDiv element no longer has any class names set.
Tests this Element to see if it matches the argument passed in.
myElement.match(match);
// returns true if #myDiv is a div. $('myDiv').match('div');
// returns true if #myDiv has the class foo and is named "bar" $('myDiv').match('.foo[name=bar]');
var el = $('myDiv'); $('myDiv').match(el); // returns true $('otherElement').match(el); // returns false
Checks all descendants of this Element for a match.
var result = myElement.contains(el);
<div id="Darth_Vader"> <div id="Luke"></div> </div>
if ($('Darth_Vader').contains($('Luke'))) alert('Luke, I am your father.'); //tan tan tannn...
Injects, or inserts, the Element at a particular place relative to the Element's children (specified by the second the argument).
myElement.inject(el[, where]);
var myFirstElement = new Element('div', {id: 'myFirstElement'}); var mySecondElement = new Element('div', {id: 'mySecondElement'}); var myThirdElement = new Element('div', {id: 'myThirdElement'});
<div id="myFirstElement"></div> <div id="mySecondElement"></div> <div id="myThirdElement"></div>
myFirstElement.inject(mySecondElement);
<div id="mySecondElement"> <div id="myFirstElement"></div> </div>
myThirdElement.inject(mySecondElement, 'top');
<div id="mySecondElement"> <div id="myThirdElement"></div> <div id="myFirstElement"></div> </div>
myFirstElement.inject(mySecondElement, 'before');
<div id="myFirstElement"></div> <div id="mySecondElement"></div>
myFirstElement.inject(mySecondElement, 'after');
<div id="mySecondElement"></div> <div id="myFirstElement"></div>
Element:adopt, Element:grab, Element:wraps
Works as Element:inject, but in reverse.
Appends the Element at a particular place relative to the Element's children (specified by the where parameter).
myElement.grab(el[, where]);
<div id="first"> <div id="child"></div> </div>
var mySecondElement = new Element('div#second'); $('first').grab(mySecondElement);
<div id="first"> <div id="child"></div> <div id="second"></div> </div>
var mySecondElement = new Element('div#second'); myFirstElement.grab(mySecondElement, 'top');
<div id="first"> <div id="second"></div> <div id="child"></div> </div>
Element:adopt, Element:inject, Element:wraps
Works like Element:grab, but allows multiple elements to be adopted and only appended at the bottom.
Inserts the passed element(s) inside the Element (which will then become the parent element).
myParent.adopt(el[, others]);
var myFirstElement = new Element('div#first'); var mySecondElement = new Element('p#second'); var myThirdElement = new Element('ul#third'); var myFourthElement = new Element('a#fourth'); var myParentElement = new Element('div#parent'); myFirstElement.adopt(mySecondElement); mySecondElement.adopt(myThirdElement, myFourthElement); myParentElement.adopt([myFirstElement, new Element('span#another')]);
<div id="parent"> <div id="first"> <p id="second"> <ul id="third"></ul> <a id="fourth"></a> </p> </div> <span id="another"></span> </div>
Element:grab, Element:inject, Element:wraps
Works like Element:grab, but replaces the element in its place, and then appends the replaced element in the location specified inside the this element.
myParent.wraps(el[, where]);
<div id="first"></div>
var mySecondElement = new Element('div#second').wraps('first');
<div id="second"> <div id="first"></div> </div>
<div id="first"></div> <div id="second"> <div id="child"></div> </div>
$('second').wraps('first');
<div id="second"> <div id="child"></div> <div id="first"></div> </div>
$('second').wraps('first', 'top');
<div id="second"> <div id="first"></div> <div id="child"></div> </div>
Works like Element:grab, but instead of accepting an id or an element, it only accepts text. A text node will be created inside this Element, in either the top or bottom position.
myElement.appendText(text[, where]);
<div id="myElement">Hey.</div>
$('myElement').appendText(' Howdy.');
<div id="myElement">Hey. Howdy.</div>
Removes the Element from the DOM.
var removedElement = myElement.dispose();
<div id="myElement"></div> <div id="mySecondElement"></div>
$('myElement').dispose();
<div id="mySecondElement"></div>
Clones the Element and returns the cloned one.
var copy = myElement.clone([contents, keepid]);
<div id="myElement">ciao</div>
// clones the Element and appends the clone after the Element. var clone = $('myElement').clone().inject('myElement','after');
<div id="myElement">ciao</div>
<div>ciao</div>
Replaces the passed Element with Element.
var element = myElement.replaces(el);
$('myNewElement').replaces($('myOldElement')); //$('myOldElement') is gone, and $('myNewElement') is in its place.
Tests the Element to see if it has the passed in className.
var result = myElement.hasClass(className);
<div id="myElement" class="testClass"></div>
$('myElement').hasClass('testClass'); // returns true
Adds the passed in class to the Element, if the Element doesnt already have it.
myElement.addClass(className);
<div id="myElement" class="testClass"></div>
$('myElement').addClass('newClass');
<div id="myElement" class="testClass newClass"></div>
Works like Element:addClass, but removes the class from the Element.
myElement.removeClass(className);
<div id="myElement" class="testClass newClass"></div>
$('myElement').removeClass('newClass');
<div id="myElement" class="testClass"></div>
Adds or removes the passed in class name to the Element, depending on whether or not it's already present.
myElement.toggleClass(className, force);
<div id="myElement" class="myClass"></div>
$('myElement').toggleClass('myClass');
<div id="myElement" class=""></div>
$('myElement').toggleClass('myClass');
<div id="myElement" class="myClass"></div>
Returns the previousSibling of the Element (excluding text nodes).
var previousSibling = myElement.getPrevious([match]);
Like Element:getPrevious, but returns a collection of all the matched previousSiblings.
As Element:getPrevious, but tries to find the nextSibling (excluding text nodes).
var nextSibling = myElement.getNext([match]);
Like Element.getNext, but returns a collection of all the matched nextSiblings.
Gets the first element that matches the passed in expression.
var firstElement = myElement.getFirst([match]);
Gets the last element that matches the passed in expression.
var lastElement = myElement.getLast([match]);
Works as Element:getPrevious, but tries to find the parentNode.
var parent = myElement.getParent([match]);
Like Element:getParent, but returns a collection of all the matched parentNodes up the tree.
Like Element:getAllPrevious but returns all Element's previous and next siblings (excluding text nodes). Returns as Elements.
var siblings = myElement.getSiblings([match]);
Returns all the Element's children (excluding text nodes). Returns as Elements.
var children = myElement.getChildren([match]);
The difference between the methods getChildren and getElements is that getChildren will only return its direct children while getElements searches for all the Elements in any depth.
Empties an Element of all its children.
myElement.empty();
<div id="myElement">
<p></p>
<span></span>
</div>
$('myElement').empty();
<div id="myElement"></div>
Removes the Element and its children from the DOM and prepares them for garbage collection.
myElement.destroy();
Reads the child inputs of the Element and generates a query string based on their values.
var query = myElement.toQueryString();
<form id="myForm" action="submit.php"> <input name="email" value="bob@bob.com" /> <input name="zipCode" value="90210" /> </form>
$('myForm').toQueryString(); // returns "email=bob@bob.com&zipCode=90210".
Returns the selected options of a select element.
var selected = mySelect.getSelected();
<select id="country-select" name="country"> <option value="US">United States</option <option value ="IT">Italy</option> </select>
$('country-select').getSelected(); // returns whatever the user selected.
This method returns an array, regardless of the multiple attribute of the select element. If the select is single, it will return an array with only one item.
Returns a single element attribute.
var myProp = myElement.getProperty(property);
<img id="myImage" src="mootools.png" title="MooTools, the compact JavaScript framework" alt="" />
var imgProps = $('myImage').getProperty('src'); // returns: 'mootools.png'.
Gets multiple element attributes.
var myProps = myElement.getProperties(properties);
<img id="myImage" src="mootools.png" title="MooTools, the compact JavaScript framework" alt="" />
var imgProps = $('myImage').getProperties('id', 'src', 'title', 'alt'); // returns: { id: 'myImage', src: 'mootools.png', title: 'MooTools, the compact JavaScript framework', alt: '' }
Sets an attribute or special property for this Element.
<img id="myImage" />
$('myImage').setProperty('src', 'mootools.png');
<img id="myImage" src="mootools.png" />
src
property for an image file, be sure to remove the width
and height
attribute (use Element.removeAttribute
). IE7, and less, set and freeze the width
and height
of an image if previously specified. Sets numerous attributes for the Element.
<img id="myImage" />
$('myImage').setProperties({ src: 'whatever.gif', alt: 'whatever dude' });
<img id="myImage" src="whatever.gif" alt="whatever dude" />
Removes an attribute from the Element.
myElement.removeProperty(property);
<a id="myAnchor" href="#" onmousedown="alert('click');"></a>
//Eww... inline JavaScript is bad! Let's get rid of it. $('myAnchor').removeProperty('onmousedown');
<a id="myAnchor" href="#"></a>
Removes numerous attributes from the Element.
myElement.removeProperties(properties);
<a id="myAnchor" href="#" title="hello world"></a>
$('myAnchor').removeProperties('id', 'href', 'title');
<a></a>
Stores an item in the Elements Storage, linked to this Element.
myElement.store(key, value);
$('element').store('someProperty', someValue);
Retrieves a value from the Elements storage.
myElement.retrieve(key[, default]);
$('element').retrieve('someProperty'); // returns someValue (see example above)
Eliminates a key from the Elements storage.
myElement.eliminate(key);
$('element').eliminate('someProperty');
This Object contains the functions that respond to the first argument passed in Element:get, Element:set and Element:erase.
Element.Properties.disabled = { get: function(){ return this.disabled; }, set: function(value){ this.disabled = !!value; this.setAttribute('disabled', !!value); } };
// gets the "disabled" property $(element).get('disabled'); // sets the "disabled" property to true, along with the attribute $(element).set('disabled', true);
Additionally, you can access these custom getters and setters using an object as the parameter for the set method.
// using set: $(divElement).set({html: '<p>Hello <em>People</em>!</p>', style: 'background:red'}); // for new Elements (works the same as set): new Element('input', {type: 'checkbox', checked: true, disabled: true});
Sets the innerHTML of the Element.
myElement.set('html', html);
<div id="myElement"></div>
$('myElement').set('html', '<div></div><p></p>');
<div id="myElement">
<div></div>
<p></p>
</div>
Returns the inner HTML of the Element.
myElement.get('html');
Sets the inner text of the Element.
myElement.set('text', text);
<div id="myElement"></div>
$('myElement').set('text', 'some text'); // the text of myElement is now 'some text'.
<div id="myElement">some text</div>
Gets the inner text of the Element.
var myText = myElement.get('text');
<div id="myElement">my text</div>
var myText = $('myElement').get('text'); // myText = 'my text'.
Returns the tag name of the Element in lower case.
var myTag = myElement.get('tag');
<img id="myImage" />
var myTag = $('myImage').get('tag'); // myTag = 'img'
Custom Type to create and easily work with IFrames.
Creates an IFrame HTML Element and extends its window and document with MooTools.
var myIFrame = new IFrame([el][, props]);
var myIFrame = new IFrame({ src: 'http://mootools.net/', styles: { width: 800, height: 600, border: '1px solid #ccc' }, events: { mouseenter: function(){ alert('Welcome aboard.'); }, mouseleave: function(){ alert('Goodbye!'); }, load: function(){ alert('The iframe has finished loading.'); } } });
The Elements class allows Element methods to work on an Elements array, as well as Array Methods.
var myElements = new Elements(elements[, options]);
$$('p').each(function(el){ el.setStyle('color', 'red'); }); // Because $$('myselector') also accepts Element methods, the below // example has the same effect as the one above. $$('p').setStyle('color', 'red');
var myElements = new Elements(['myElementID', $('myElement'), 'myElementID2', document.getElementById('myElementID3')]);
Adds the items of the collection to this Elements array, and return the this array.
elements.append(collection);
Adds the element, or array of Elements, to this Elements array, and returns a new Elements array.
var newElements = elements.concat(element[, list, id, ...]]);
Removes every item from the Elements array, and the empty array.
elements.empty();
Elements.empty
does not destroy the elements inside. As best practice, always destroy your elements if they're no longer in use. For example:
$$('div').destroy().empty();
Filters a collection of elements by a given css selector, or filtering function like Array:filter.
var filteredElements = elements.filter(selector);
Adds the element, or elements, to the end of this Elements array and returns the length of the array.
var length = elements.push(element[, id, ...]]);
Adds the element, or elements, to the front of this Elements array and returns the length of the array.
var length = elements.unshift(element[, id, ...]]);
This method has been deprecated. Use Element:contains instead.
var myElement = document.id('element1'); var myElement2 = document.id('element2'); myElement !== myElement2 && myElement.contains(element2); // could be implemented as: Element.implement('hasChild', function(element){ return this !== element && this.contains(element); });
This method has been deprecated. Use Element:inject instead.
This method has been deprecated. Use Element:inject instead.
This method has been deprecated. Use Element:inject instead.
This method has been deprecated. Use Element:inject instead.
This method has been deprecated. Use Element:inject instead.
This method has been deprecated. Use Element:grab instead.
This method has been deprecated. Use Element:grab instead.
This method has been deprecated. Use Element:grab instead.
This method has been deprecated. Use Element:grab instead.
This method has been deprecated. Use Element:grab instead.
This method has been deprecated. Use Elements:append instead.
© Linux.ria.ua, 2008-2024 |