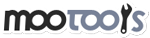
Slick is the selector engine used by MooTools. It supports many CSS3 selectors and more!
Reverse Combinators redirect the flow of selectors and combinators. Slick implements these by prepending !
to a selector or combinator.
document.getElement('p ! div') // A <div> that is an ancestor of a <p> document.getElement('p !> div') // A <div> that is a direct parent of a <p> document.getElement('.foo !+ p') // Gets the previous adjacent <p> sibling
Reverse Combinators are used internally by MooTools for many of our traversal methods. They offer an extremely concise and powerful alternative to traversal methods like getParent()
.
definePseudo allows you to create your own custom pseudo selectors.
Slick.definePseudo('display', function(value){ return Element.getStyle(this, 'display') == value; }); <div style="display: none">foo</div> <div style="display: block">bar</div> $$(':display(block)'); // Will return the block element Slick.definePseudo('my-custom-pseudo', function(){ // 'this' is the node to check return Element.retrieve(this, 'something-custom').isAwesome; }); $$(':my-custom-pseudo') // Will return the first <p> tag that is awesome
Gets the next siblings.
$$('p.foo ~') // Gets all next siblings of <p class="foo"> $$('p.foo ~ blockquote') // Gets every <blockquote> with a <p class="foo"> sibling somewhere *before* it
Gets the previous siblings.
$$('p.foo !~') // Gets all previous siblings of <p class="foo"> $$('p.foo !~ blockquote') // Gets every <blockquote> with a <p class="foo"> sibling somewhere *after* it
Gets all siblings.
$$('p.foo ~~') // Gets all previous and next siblings of <p class="foo"> $$('p.foo ~~ blockquote') // Gets every <blockquote> with a <p class="foo"> sibling before OR after it
Gets the first child of an element.
$$('p.foo ^') // Gets the first child of <p class="foo"> $$('p.foo ^ strong') // Gets every <strong> that is the first element child of a <p class="foo">
Gets the last child of an element.
$$('p.foo !^') // Gets the last child of <p class="foo"> $$('p.foo !^ strong') // Gets every <strong> that is the last element child of a <p class="foo">
Matches all Elements that are checked.
$$(':checked') $('myForm').getElements('input:checked');
Matches all Elements that are enabled.
$$(':enabled') $('myElement').getElements(':enabled');
Matches all elements which are empty.
$$(':empty');
Matches all the Elements which contains the text.
$$('p:contains("find me")');
Gets the element in focus.
$$(':focus'); // Gets the element in focus
Matches all elements that do not match the selector.
Note: The Slick implementation of the :not
pseudoClass is a superset of the standard. i.e. it is more advanced than the specification.
$$(':not(div.foo)'); // all elements except divs with class 'foo' $$('input:not([type="submit"])'); // all inputs except submit buttons myElement.getElements(':not(a)'); $$(':not(ul li)');
Matches every nth child.
Nth Expression:
':nth-child(nExpression)'
<span id="i1"></span> <span id="i2"></span> <span id="i3"></span> <span id="i4"></span> <span id="i5"></span> $$(':nth-child(1)'); //Returns Element #i1. $$(':nth-child(2n)'); //Returns Elements #i2 and #i4. $$(':nth-child(2n+1)') //Returns Elements #i1, #i3 and #i5. $$(':nth-child(3n+2)') //Returns Elements #i2 and #i5.
Every Odd Child (same as 2n+1):
':nth-child(odd)'
Every Even Child (same as 2n):
':nth-child(even)'
This selector respects the w3c specifications, so it has 1 as its first index, not 0. Therefore nth-child(odd) will actually select the even children, if you think in zero-based indexes.
Matches every nth child, starting from the last child.
Nth Expression:
':nth-last-child(nExpression)'
<span id="i1"></span> <span id="i2"></span> <span id="i3"></span> <span id="i4"></span> <span id="i5"></span> $$(':nth-last-child(1)'); //Returns Element #i5. $$(':nth-last-child(2n)'); //Returns Elements #i2 and #i4. $$(':nth-last-child(2n+1)') //Returns Elements #i1, #i3 and #i5. $$(':nth-last-child(3n+2)') //Returns Elements #i1 and #i4.
Every Odd Child (same as 2n+1):
':nth-last-child(odd)'
Every Even Child (same as 2n):
':nth-last-child(even)'
This selector respects the w3c specifications, so it has 1 as its first index, not 0. Therefore nth-last-child(odd) will actually select the even last-children, if you think in zero-based indexes.
Matches every even child.
$$('td:even');
This selector is not part of the w3c specification, therefore its index starts at 0. This selector is highly recommended over nth-child(even), as this will return the real even children.
Matches every odd child.
$$('td:odd');
This selector is not part of the w3c specification, therefore its index starts at 0. This selector is highly recommended over nth-child(odd), as this will return the real odd children.
Matches the node at the specified index
$$('p:index(2)'); // Gets the third <p> tag.
This is zero-indexed.
Matches the first child.
':first-child'
$$('td:first-child');
Matches the last child.
':last-child'
$$('td:last-child');
Matches an only child of its parent Element.
':only-child'
$$('td:only-child');
© Linux.ria.ua, 2008-2024 |