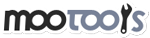
Attaches an event listener to a DOM element.
myElement.addEvent(type, fn);
<div id="myElement">Click me.</div>
$('myElement').addEvent('click', function(){ alert('clicked!'); });
Works as Element.addEvent, but instead removes the specified event listener.
myElement.removeEvent(type, fn);
var destroy = function(){ alert('Boom: ' + this.id); } // this refers to the Element. $('myElement').addEvent('click', destroy); //later... $('myElement').removeEvent('click', destroy);
var destroy = function(){ alert('Boom: ' + this.id); } var boundDestroy = destroy.bind($('anotherElement')); $('myElement').addEvent('click', boundDestroy); //later... $('myElement').removeEvent('click', destroy); // this won't remove the event. $('myElement').removeEvent('click', destroy.bind($('anotherElement')); // this won't remove the event either. $('myElement').removeEvent('click', boundDestroy); // the correct way to remove the event.
The same as Element:addEvent, but accepts an object to add multiple events at once.
myElement.addEvents(events);
$('myElement').addEvents({ mouseover: function(){ alert('mouseover'); }, click: function(){ alert('click'); } });
Removes all events of a certain type from an Element. If no argument is passed, removes all events of all types.
myElements.removeEvents([events]);
var myElement = $('myElement'); myElement.addEvents({ mouseover: function(){ alert('mouseover'); }, click: function(){ alert('click'); } }); myElement.addEvent('click', function(){ alert('clicked again'); }); myElement.addEvent('click', function(){ alert('clicked and again :('); }); //addEvent will keep appending each function. //Unfortunately for the visitor, there will be three alerts they'll have to click on. myElement.removeEvents('click'); // saves the visitor's finger by removing every click event.
Executes all events of the specified type present in the Element.
myElement.fireEvent(type[, args[, delay]]);
// fires all the added 'click' events and passes the Element 'anElement' after one second $('myElement').fireEvent('click', $('anElement'), 1000);
Clones all events from an Element to this Element.
myElement.cloneEvents(from[, type]);
var myElement = $('myElement'); var myClone = myElement.clone().cloneEvents(myElement); // clones the element and its events
You can add additional custom events by adding properties (objects) to the Element.Events Object
The Element.Events.yourProperty (object) can have:
Element.Events.shiftclick = { base: 'click', // the base event type condition: function(event){ //a function to perform additional checks return (event.shift == true); // this means the event is free to fire } }; $('myInput').addEvent('shiftclick', function(event){ log('the user clicked the left mouse button while holding the shift key'); });
If you use the condition option you NEED to specify a base type, unless you plan to overwrite a native event. (highly unrecommended: use only when you know exactly what you're doing).
This event fires when the mouse enters the area of the DOM Element and will not be fired again if the mouse crosses over children of the Element (unlike mouseover).
$('myElement').addEvent('mouseenter', myFunction);
This event fires when the mouse leaves the area of the DOM Element and will not be fired if the mouse crosses over children of the Element (unlike mouseout).
$('myElement').addEvent('mouseleave', myFunction);
This event fires when the mouse wheel is rotated;
$('myElement').addEvent('mousewheel', myFunction);
mouseenter
and mouseleave
events are supported natively by Internet Explorer, Opera 11, and Firefox 10. MooTools will only add the custom events if necessary.
This custom event just redirects DOMMouseScroll (Mozilla) to mousewheel (Opera, Internet Explorer), making it work across browsers.
This is an object with all known DOM event types, like click, mouseover, load, etc.
Each event type has a value, possible values are 0
(undefined
, null
), 1
, and 2
.
By default it is undefined. In this case you can add events, but you should manually fire them.
element.addEvent('pizza', fn); element.fireEvent('pizza', 'yum!');
The event is not actually added to the DOM, but is only registered in a JS object.
The second case is if the value is 1. This time the object is attached to the DOM. Usually by element.addEventListener, or element.attachEvent in older versions of IE. You can still use element.fireEvent('load')
to manually fire events.
The final case is if the value is 2. This is the same as case 1. The only difference is that the event object, containing interesting data, is wrapped and normalized by event wrapper (DOMEvent). This is the most used variant, for mouse events (like click) and keyboard events.
The reason to differentiate between 1 and 2 is that 1 is usually used for events that don't have interesting data like: onload
, onscroll
, and onresize
, or it's more performant. The latter two, for example, are fired frequently.
Not all events are supported by MooTools' Element Events API because of edge use cases or new events supported by the browser. To add support for a native event, just augment the Element.NativeEvents
object with the key and appropriate key value (use the above). For example to add popstate
support in your application:
Element.NativeEvents.popstate = 2; // Now element.addEvent('popstate', fn); will work everywhere
© Linux.ria.ua, 2008-2024 |