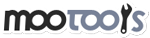
Документация
на русском v1.2.5 Документацитя по MooTools 1.4.5 Обсуждение Установить себе Благодарности
на русском v1.2.5 Документацитя по MooTools 1.4.5 Обсуждение Установить себе Благодарности
The base Class of the MooTools framework.
var MyClass = new Class(properties);
The methods of This Class that have the same name as the Extends Class, will have a parent property, that allows you to call the other overridden method.
Implements is similar to Extends, except that it overrides properties without inheritance. Useful when implementing a default set of properties in multiple Classes.
var Cat = new Class({ initialize: function(name){ this.name = name; } }); var myCat = new Cat('Micia'); alert(myCat.name); //alerts 'Micia' var Cow = new Class({ initialize: function(){ alert('moooo'); } }); var Effie = new Cow($empty); //Will not alert 'moooo', because the initialize method is overridden by the $empty function.
var Animal = new Class({ initialize: function(age){ this.age = age; } }); var Cat = new Class({ Extends: Animal, initialize: function(name, age){ this.parent(age); //will call initalize of Animal this.name = name; } }); var myCat = new Cat('Micia', 20); alert(myCat.name); //Alerts 'Micia'. alert(myCat.age); //Alerts 20.
var Animal = new Class({ initialize: function(age){ this.age = age; } }); var Cat = new Class({ Implements: Animal, setName: function(name){ this.name = name } }); var myAnimal = new Cat(20); myAnimal.setName('Micia'); alert(myAnimal.name); //Alerts 'Micia'.
Implements the passed in properties into the base Class prototypes, altering the base Class. The same as creating a new Class with the Implements property, but handy when you need to modify existing classes.
MyClass.implement(properties);
var Animal = new Class({ initialize: function(age){ this.age = age; } }); Animal.implement({ setName: function(name){ this.name = name; } }); var myAnimal = new Animal(20); myAnimal.setName('Micia'); alert(myAnimal.name); //alerts 'Micia'
© Linux.ria.ua, 2008-2024 |