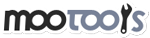
Документация
на русском v1.2.5 Документацитя по MooTools 1.4.5 Обсуждение Установить себе Благодарности
на русском v1.2.5 Документацитя по MooTools 1.4.5 Обсуждение Установить себе Благодарности
The slide effect slides an Element in horizontally or vertically. The contents will fold inside.
var myFx = new Fx.Slide(element[, options]);
//Hides the Element, then brings it back in with toggle and finally alerts //when complete: var mySlide = new Fx.Slide('container').hide().toggle().chain(function(){ alert(mySlide.open); //Alerts true. });
Slides the Element in view horizontally or vertically.
myFx.slideIn([mode]);
var myFx = new Fx.Slide('myElement').slideOut().chain(function(){ this.show().slideIn('horizontal'); });
Slides the Element out of view horizontally or vertically.
myFx.slideOut([mode]);
var myFx = new Fx.Slide('myElement', { mode: 'horizontal', //Due to inheritance, all the [Fx][] options are available. onComplete: function(){ alert('Poof!'); } //The mode argument provided to slideOut overrides the option set. }).slideOut('vertical');
Slides the Element in or out, depending on its state.
myFx.toggle([mode]);
var myFx = new Fx.Slide('myElement', { duration: 1000, transition: Fx.Transitions.Pow.easeOut }); //Toggles between slideIn and slideOut twice: myFx.toggle().chain(myFx.toggle);
Hides the Element without a transition.
myFx.hide([mode]);
var myFx = new Fx.Slide('myElement', { duration: 'long', transition: Fx.Transitions.Bounce.easeOut }); //Automatically hides and then slies in "myElement": myFx.hide().slideIn();
Shows the Element without a transition.
myFx.show([mode]);
var myFx = new Fx.Slide('myElement', { duration: 1000, transition: Fx.Transitions.Bounce.easeOut }); //Slides "myElement" out. myFx.slideOut().chain(function(){ //Waits one second, then the Element appears without transition. this.show.delay(1000, this); });
See Element.Properties.
Sets a default Fx.Slide instance for an element. Gets the previously setted Fx.Slide instance or a new one with default options.
el.set('slide'[, options]);
el.set('slide', {duration: 'long', transition: 'bounce:out'}); el.slide('in');
el.get('slide');
el.set('slide', {duration: 'long', transition: 'bounce:out'}); el.slide('in'); el.get('slide'); //the Fx.Slide instance
Custom Native to allow all of its methods to be used with any DOM element via the dollar function $.
Slides this Element in view.
myElement.slide(how);
$('myElement').slide('hide').slide('in');
© Linux.ria.ua, 2008-2024 |