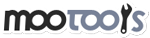
на русском v1.2.5 Документацитя по MooTools 1.4.5 Обсуждение Установить себе Благодарности
Function Methods.
Base function for creating functional closures which is used by all other Function prototypes.
var createdFunction = myFunction.create([options]);
var myFunction = function(){ alert("I'm a function. :D"); }; var mySimpleFunction = myFunction.create(); //Just a simple copy. var myAdvancedFunction = myFunction.create({ //When called, this function will attempt. Аргументы: [0,1,2,3], attempt: true, delay: 1000, bind: myElement });
Returns a closure with arguments and bind.
var newFunction = myFunction.pass([args[, bind]]);
var myFunction = function(){ var result = 'Passed: '; for (var i = 0, l = arguments.length; i < l; i++){ result += (arguments[i] + ' '); } return result; } var myHello = myFunction.pass('hello'); var myItems = myFunction.pass(['peach', 'apple', 'orange']); //Later in the code, the functions can be executed: alert(myHello()); //Passes "hello" to myFunction. alert(myItems()); //Passes the array of items to myFunction.
Tries to execute the function.
var result = myFunction.attempt([args[, bind]]);
null
if an exception is thrown.var myObject = { 'cow': 'moo!' }; var myFunction = function(){ for (var i = 0; i < arguments.length; i++){ if(!this[arguments[i]]) throw('doh!'); } }; var result = myFunction.attempt(['pig', 'cow'], myObject); //result = null
Changes the scope of this
within the target function to refer to the bind parameter.
myFunction.bind([bind[, args[, evt]]]);
function myFunction(){ //Note that 'this' here refers to window, not an element. //The function must be bound to the element we want to manipulate. this.setStyle('color', 'red'); }; var myBoundFunction = myFunction.bind(myElement); myBoundFunction(); //This will make myElement's text red.
Changes the scope of this
within the target function to refer to the bind parameter. It also makes "space" for an event.
This allows the function to be used in conjunction with Element:addEvent and arguments.
myFunction.bindWithEvent([bind[, args[, evt]]]);
function myFunction(e, add){ //Note that 'this' here refers to window, not an element. //We'll need to bind this function to the element we want to alter. this.setStyle('top', e.client.x + add); }; $(myElement).addEvent('click', myFunction.bindWithEvent(myElement, 100)); //When clicked, the element will move to the position of the mouse + 100.
Delays the execution of a function by a specified duration.
var timeoutID = myFunction.delay([delay[, bind[, args]]]);
var myFunction = function(){ alert('moo! Element id is: ' + this.id); }; //Wait 50 milliseconds, then call myFunction and bind myElement to it. myFunction.delay(50, myElement); //Alerts: 'moo! Element id is: ... ' //An anonymous function which waits a second and then alerts. (function(){ alert('one second later...'); }).delay(1000);
Executes a function in the specified intervals of time. Periodic execution can be stopped using the $clear function.
var intervalID = myFunction.periodical([period[, bind[, args]]]);
var Site = { counter: 0 }; var addCount = function(){ this.counter++; }; addCount.periodical(1000, Site); //Will add the number of seconds at the Site.
Runs the Function with specified arguments and binding. The same as apply but reversed and with support for a single argument.
var myFunctionResult = myFunction.run(args[, bind]);
var myFn = function(a, b, c){ return a + b + c; } var myArgs = [1,2,3]; myFn.run(myArgs); //Возвращает: 6
var myFn = function(a, b, c) { return a + b + c + this; } var myArgs = [1,2,3]; myFn.run(myArgs, 6); //Возвращает: 12
© Linux.ria.ua, 2008-2024 |