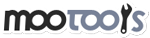
Документация
на русском v1.2.5 Документацитя по MooTools 1.4.5 Обсуждение Установить себе Благодарности
на русском v1.2.5 Документацитя по MooTools 1.4.5 Обсуждение Установить себе Благодарности
MooTools Event Methods.
new Event([event[, win]]);
extended
with $.$('myLink').addEvent('keydown', function(event){ //The passed event parameter is already an instance of the Event class. alert(event.key); //Returns the lowercase letter pressed. alert(event.shift); //Returns true if the key pressed is shift. if (event.key == 's' && event.control) alert('Document saved.'); //Executes if the user hits Ctr+S. });
Stop an Event from propagating and also executes preventDefault.
myEvent.stop();
<a id="myAnchor" href="http://google.com/">Visit Google.com</a>
$('myAnchor').addEvent('click', function(event){ event.stop(); //Prevents the browser from following the link. this.set('text', "Where do you think you're going?"); //'this' is Element that fires the Event. (function(){ this.set('text', "Instead visit the Blog.").set('href', 'http://blog.mootools.net'); }).delay(500, this); });
Cross browser method to stop the propagation of an event (this stops the event from bubbling up through the DOM).
myEvent.stopPropagation();
"#myChild" does not cover the same area as myElement. Therefore, the 'click' differs from parent and child depending on the click location:
<div id="myElement"> <div id="myChild"></div> </div>
$('myElement').addEvent('click', function(){ alert('click'); return false; // equivalent to stopPropagation. }); $('myChild').addEvent('click', function(event){ event.stopPropagation(); // this will prevent the event to bubble up, and fire the parent's click event. });
Cross browser method to prevent the default action of the event.
myEvent.preventDefault();
<form> <input id="myCheckbox" type="checkbox" /> </form>
$('myCheckbox').addEvent('click', function(event){ event.preventDefault(); //Will prevent the checkbox from being "checked". });
Additional Event key codes can be added by adding properties to the Event.Keys Hash.
Event.Keys.shift = 16; $('myInput').addEvent('keydown', function(event){ if (event.key == "shift") alert("You pressed shift."); });
© Linux.ria.ua, 2008-2024 |