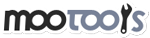
на русском v1.2.5 Документацитя по MooTools 1.4.5 Обсуждение Установить себе Благодарности
Следующие ниже функции рассматриваются как методы Window.
У функции dollar двойная цель: Получить элемент по его идентификатору ID, а также «захватить» все методы Element элемента в Internet Explorer.
var myElement = $(el);
var myElement = $('myElement');
var div = document.getElementById('myElement'); div = $(div); //Элемент со всеми применяемыми к нему медотами Element.
Выбирает и расширяет элементы DOM. Элементы массивов, возвращенных с помощью функции $$, будут также содержать все применяемые к ним методы Element.
var myElements = $$(aTag[, anElement[, Elements[, ...]);
$$('a'); //Возвращает все элементы с тегом <a>. $$('a', 'b'); //Возвращает все элементы с тегами <a> и <b>.
$$('#myElement'); //Возвращает массив, содержащий только элемент с идентификатором 'myElement'. $$('#myElement a.myClass'); //Возвращает массив всех элементов с тегом <a> и с классом 'myClass' в пределах элемента DOM с идентификатором 'myElement'.
//Создает массив всех элементов и селекторов, принятых в качестве аргументов. $$(myelement1, myelement2, 'a', '#myid, #myid2, #myid3', document.getElementsByTagName('div'));
Custom Native to allow all of its methods to be used with any extended DOM Element.
Creates a new Element of the type passed in.
var myEl = new Element(element[, properties]);
var myAnchor = new Element('a', { 'href': 'http://mootools.net', 'class': 'myClass', 'html': 'Click me!', 'styles': { 'display': 'block', 'border': '1px solid black' }, 'events': { 'click': function(){ alert('clicked'); }, 'mouseover': function(){ alert('mouseovered'); } } });
Gets the first descendant element whose tag name matches the tag provided. If Selectors is included, CSS selectors may also be passed.
var myElement = myElement.getElement(tag);
var firstDiv = $(document.body).getElement('div');
Collects all decedent elements whose tag name matches the tag provided. If Selectors is included, CSS selectors may also be passed.
var myElements = myElement.getElements(tag);
var allAnchors = $(document.body).getElements('a');
Gets the element with the specified id found inside the current Element.
var myElement = anElement.getElementById(id);
var myChild = $('myParent').getElementById('myChild');
This is a "dynamic arguments" method. Properties passed in can be any of the 'set' properties in the Element.Properties Hash.
myElement.set(arguments);
$('myElement').set('text', 'text goes here'); $('myElement').set('class', 'active'); //The 'styles' property passes the object to Element:setStyles. var body = $(document.body).set('styles', { 'font': '12px Arial', 'color': 'blue' });
var myElement = $('myElement').set({ //The 'styles' property passes the object to Element:setStyles. 'styles': { 'font': '12px Arial', 'color': 'blue', 'border': '1px solid #f00' }, //The 'events' property passes the object to Element:addEvents. 'events': { 'click': function(){ alert('click'); }, 'mouseover': function(){ this.addClass('over') } }, //Any other property uses Element:setProperty. 'id': 'documentBody' });
This is a "dynamic arguments" method. Properties passed in can be any of the 'get' properties in the Element.Properties Hash.
myElement.get(property);
var tag = $('myDiv').get('tag'); //Returns "div".
var id = $('myDiv').get('id'); //Returns "myDiv". var value = $('myInput').get('value'); //Returns the myInput element's value.
This is a "dynamic arguments" method. Properties passed in can be any of the 'erase' properties in the Element.Properties Hash.
myElement.erase(property);
$('myDiv').erase('id'); //Removes the id from myDiv. $('myDiv').erase('class'); //myDiv element no longer has any class names set.
Tests this Element to see if it matches the argument passed in.
myElement.match(match);
//Returns true if #myDiv is a div. $('myDiv').match('div');
//Returns true if #myDiv has the class foo and is named "bar" $('myDiv').match('.foo[name=bar]');
var el = $('myDiv'); $('myDiv').match(el); //Returns true $('otherElement').match(el); //Returns false
Injects, or inserts, the Element at a particular place relative to the Element's children (specified by the second the argument).
myElement.inject(el[, where]);
var myFirstElement = new Element('div', {id: 'myFirstElement'}); var mySecondElement = new Element('div', {id: 'mySecondElement'}); var myThirdElement = new Element('div', {id: 'myThirdElement'});
<div id="myFirstElement"></div> <div id="mySecondElement"></div> <div id="myThirdElement"></div>
myFirstElement.inject(mySecondElement);
<div id="mySecondElement"> <div id="myFirstElement"></div> </div>
myThirdElement.inject(mySecondElement, 'top');
<div id="mySecondElement"> <div id="myThirdElement"></div> <div id="myFirstElement"></div> </div>
myFirstElement.inject(mySecondElement, 'before');
<div id="myFirstElement"></div> <div id="mySecondElement"></div>
myFirstElement.inject(mySecondElement, 'after');
<div id="mySecondElement"></div> <div id="myFirstElement"></div>
Element:adopt, Element:grab, Element:wraps
Works as Element:inject, but in reverse.
Appends the Element at a particular place relative to the Element's children (specified by the where parameter).
myElement.grab(el[, where]);
var myFirstElement = new Element('div', {id: 'myFirstElement'}); var mySecondElement = new Element('div', {id: 'mySecondElement'}); myFirstElement.grab(mySecondElement);
<div id="myFirstElement"> <div id="mySecondElement"></div> </div>
Element:adopt, Element:inject, Element:wraps
Works like Element:grab, but allows multiple elements to be adopted.
Inserts the passed element(s) inside the Element (which will then become the parent element).
myParent.adopt(el[, others]);
var myFirstElement = new Element('div', {id: 'myFirstElement'}); var mySecondElement = new Element('a', {id: 'mySecondElement'}); var myThirdElement = new Element('ul', {id: 'myThirdElement'}); myParent.adopt(myFirstElement); myParent2.adopt(myFirstElement, 'mySecondElement'); myParent3.adopt([myFirstElement, mySecondElement, myThirdElement]);
<div id="myParent"> <div id="myFirstElement" /> </div> <div id="myParent2"> <div id="myFirstElement" /> <a /> </div> <div id="myParent3"> <div id="myFirstElement" /> <a /> <ul /> </div>
Element:grab, Element:inject, Element:wraps
Works like Element:grab, but instead of moving the grabbed element from its place, this method moves this Element around its target.
The Element is moved to the position of the passed element and becomes the parent.
myParent.wraps(el[, where]);
<div id="myFirstElement"></div>
var mySecondElement = new Element('div', {id: 'mySecondElement'}); mySecondElement.wraps($('myFirstElement'));
<div id="mySecondElement"> <div id="myFirstElement"></div> </div>
Works like Element:grab, but instead of accepting an id or an element, it only accepts text. A text node will be created inside this Element, in either the top or bottom position.
myElement.appendText(text);
<div id="myElement">Hey.</div>
$('myElement').appendText(' Howdy.');
<div id="myElement">Hey. Howdy.</div>
Removes the Element from the DOM.
var removedElement = myElement.dispose();
<div id="myElement"></div> <div id="mySecondElement"></div>
$('myElement').dispose();
<div id="mySecondElement"></div>
Clones the Element and returns the cloned one.
var copy = myElement.clone([contents, keepid]);
<div id="myElement"></div>
//Clones the Element and appends the clone after the Element. var clone = $('myElement').clone().injectAfter('myElement');
<div id="myElement">ciao</div>
<div>ciao</div>
Replaces the Element with an Element passed.
var element = myElement.replaces(el);
$('myNewElement').replaces($('myOldElement')); //$('myOldElement') is gone, and $('myNewElement') is in its place.
Tests the Element to see if it has the passed in className.
var result = myElement.hasClass(className);
<div id="myElement" class="testClass"></div>
$('myElement').hasClass('testClass'); //returns true
Adds the passed in class to the Element, if the Element doesnt already have it.
myElement.addClass(className);
<div id="myElement" class="testClass"></div>
$('myElement').addClass('newClass');
<div id="myElement" class="testClass newClass"></div>
Works like Element:addClass, but removes the class from the Element.
myElement.removeClass(className);
<div id="myElement" class="testClass newClass"></div>
$('myElement').removeClass('newClass');
<div id="myElement" class="testClass"></div>
Adds or removes the passed in class name to the Element, depending on whether or not it's already present.
myElement.toggleClass(className);
<div id="myElement" class="myClass"></div>
$('myElement').toggleClass('myClass');
<div id="myElement" class=""></div>
$('myElement').toggleClass('myClass');
<div id="myElement" class="myClass"></div>
Returns the previousSibling of the Element (excluding text nodes).
var previousSibling = myElement.getPrevious([match]);
Like Element:getPrevious, but returns a collection of all the matched previousSiblings.
As Element:getPrevious, but tries to find the nextSibling (excluding text nodes).
var nextSibling = myElement.getNext([match]);
Like Element.getNext, but returns a collection of all the matched nextSiblings.
Works as Element:getPrevious, but tries to find the firstChild (excluding text nodes).
var firstElement = myElement.getFirst([match]);
Works as Element:getPrevious, but tries to find the lastChild.
var lastElement = myElement.getLast([match]);
Works as Element:getPrevious, but tries to find the parentNode.
var parent = myElement.getParent([match]);
Like Element:getParent, but returns a collection of all the matched parentNodes up the tree.
Returns all the Element's children (excluding text nodes). Returns as Elements.
var children = myElement.getChildren([match]);
Checks all descendants of this Element for a match.
var result = myElement.hasChild(el);
<div id="Darth_Vader"> <div id="Luke"></div> </div>
if ($('Darth_Vader').hasChild('Luke')) alert('Luke, I am your father.'); // tan tan tannn...
Empties an Element of all its children.
myElement.empty();
<div id="myElement">
<p></p>
<span></span>
</div>
$('myElement').empty();
<div id="myElement"></div>
Empties an Element of all its children, removes and garbages the Element. Useful to clear memory before the pageUnload.
myElement.destroy();
Reads the child inputs of the Element and generates a query string based on their values.
var query = myElement.toQueryString();
<form id="myForm" action="submit.php"> <input name="email" value="bob@bob.com" /> <input name="zipCode" value="90210" /> </form>
$('myForm').toQueryString(); //Returns "email=bob@bob.com&zipCode=90210".
Returns the selected options of a select element.
var selected = mySelect.getSelected();
<select id="country-select" name="country"> <option value="US">United States</option <option value ="IT">Italy</option> </select>
$('country-select').getSelected(); //Returns whatever the user selected.
This method returns an array, regardless of the multiple attribute of the select element. If the select is single, it will return an array with only one item.
Returns a single element attribute.
var myProp = myElement.getProperty(property);
<img id="myImage" src="mootools.png" title="MooTools, the compact JavaScript framework" alt="" />
var imgProps = $('myImage').getProperty('src'); //Возвращает: 'mootools.png'.
Gets multiple element attributes.
var myProps = myElement.getProperties(properties);
<img id="myImage" src="mootools.png" title="MooTools, the compact JavaScript framework" alt="" />
var imgProps = $('myImage').getProperties('id', 'src', 'title', 'alt'); //Возвращает: { id: 'myImage', src: 'mootools.png', title: 'MooTools, the compact JavaScript framework', alt: '' }
Sets an attribute or special property for this Element.
<img id="myImage" />
$('myImage').setProperty('src', 'mootools.png');
<img id="myImage" src="mootools.png" />
Sets numerous attributes for the Element.
<img id="myImage" />
$('myImage').setProperties({ src: 'whatever.gif', alt: 'whatever dude' });
<img id="myImage" src="whatever.gif" alt="whatever dude" />
Removes an attribute from the Element.
myElement.removeProperty(property);
<a id="myAnchor" href="#" onmousedown="alert('click');"></a>
//Eww... inline JavaScript is bad! Let's get rid of it. $('myAnchor').removeProperty('onmousedown');
<a id="myAnchor" href="#"></a>
Removes numerous attributes from the Element.
myElement.removeProperties(properties);
<a id="myAnchor" href="#" title="hello world"></a>
$('myAnchor').removeProperties('id', 'href', 'title');
<a></a>
Stores an item in the Elements Storage, linked to this Element.
myElement.store(key, value);
$('element').store('someProperty', someValue);
Retrieves a value from the Elements storage.
myElement.retrieve(key[, default]);
$('element').retrieve('someProperty'); // returns someValue (see example above)
This Hash contains the functions that respond to the first argument passed in Element:get, Element:set and Element:erase.
Element.Properties.disabled = { get: function(){ return this.disabled; } set: function(value){ this.disabled = !!value; this.setAttribute('disabled', !!value); } };
//Gets the "disabled" property. $(element).get('disabled'); //Sets the "disabled" property to true, along with the attribute. $(element).set('disabled', true);
Automatically returns the element for setters.
Additionally, you can access these custom getters and setters using an object as the parameter for the set method.
//Using set: $(divElement).set({html: '<p>Hello <em>People</em>!</p>', style: 'background:red'}); //For new Elements (works the same as set): new Element('input', {type: 'checkbox', checked: true, disabled: true});
Sets the innerHTML of the Element.
myElement.set('html', [htmlString[, htmlString2[, htmlString3[, ..]]]);
<div id="myElement"></div>
$('myElement').set('html', '<div></div>', '<p></p>');
<div id="myElement">
<div></div>
<p></p>
</div>
Returns the inner HTML of the Element.
myElement.get('html');
Sets the inner text of the Element.
myElement.set('text', text);
<div id="myElement"></div>
$('myElement').set('text', 'some text'); //The text of myElement is now 'some text'.
<div id="myElement">some text</div>
Gets the inner text of the Element.
var myText = myElement.get('text');
<div id="myElement">my text</div>
var myText = $('myElement').get('text'); //myText = 'my text'.
Returns the tag name of the Element in lower case.
var myTag = myElement.get('tag');
<img id="myImage" />
var myTag = $('myImage').get('tag'); // myTag = 'img'.
Custom Native to create and easily work with IFrames.
Creates an IFrame HTML Element and extends its window and document with MooTools.
var myIFrame = new IFrame([el][, props]);
var myIFrame = new IFrame({ src: 'http://mootools.net/', styles: { width: 800, height: 600, border: '1px solid #ccc' }, events: { mouseenter: function(){ alert('Welcome aboard.'); }, mouseleave: function(){ alert('Goodbye!'); }, load: function(){ alert('The iframe has finished loading.'); } } });
The Elements class allows Element methods to work on an Elements array, as well as Array Methods.
var myElements = new Elements(elements[, options]);
$$('p').each(function(el){ el.setStyle('color', 'red'); }); //Because $$('myselector') also accepts Element methods, the below //example has the same effect as the one above. $$('p').setStyle('color', 'red');
var myElements = new Elements(['myElementID', $('myElement'), 'myElementID2', document.getElementById('myElementID3')]);
Filters a collection of elements by a given tag name. If Selectors is included, this method will be able to filter by any selector. It also works like Array:filter, by filtering collection of elements with a function.
var filteredElements = elements.filter(selector);
© Linux.ria.ua, 2008-2024 |