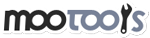
на русском v1.2.5 Документацитя по MooTools 1.4.5 Обсуждение Установить себе Благодарности
A collection of the String Object prototype methods.
Searches for a match between the string and a regular expression. For more information see MDC Regexp:test.
myString.test(regex[,params]);
true
, if a match for the regular expression is found in this string.false
if is not found"I like cookies".test("cookie"); //returns true "I like cookies".test("COOKIE", "i"); //returns true (ignore case) "I like cookies".test("cake"); //returns false
Checks to see if the string passed in is contained in this string. If the separator parameter is passed, will check to see if the string is contained in the list of values separated by that parameter.
myString.contains(string[, separator]);
true
if the string is contained in this stringfalse
if not.'a bc'.contains('bc'); //returns true 'a b c'.contains('c', ' '); //returns true 'a bc'.contains('b', ' '); //returns false
Trims the leading and trailing spaces off a string.
myString.trim();
" i like cookies ".trim(); //"i like cookies"
Removes all extraneous whitespace from a string and trims it (String:trim).
myString.clean();
" i like cookies \n\n".clean(); //returns "i like cookies"
Converts a hyphenated string to a camelcased string.
myString.camelCase();
"I-like-cookies".camelCase(); //returns "ILikeCookies"
Converts a camelcased string to a hyphenated string.
myString.hyphenate();
"ILikeCookies".hyphenate(); //returns "I-like-cookies"
Converts the first letter of each word in a string to uppercase.
myString.capitalize();
"i like cookies".capitalize(); //returns "I Like Cookies"
Escapes all regular expression characters from the string.
myString.escapeRegExp();
'animals.sheep[1]'.escapeRegExp(); //returns 'animals\.sheep\[1\]'
Parses this string and returns a number of the specified radix or base.
myString.toInt([base]);
"4em".toInt(); //returns 4 "10px".toInt(); //returns 10
Parses this string and returns a floating point number.
myString.toFloat();
"95.25%".toFloat(); //returns 95.25 "10.848".toFloat(); //returns 10.848
Converts a hexidecimal color value to RGB. Input string must be in one of the following hexidecimal color formats (with or without the hash). '#ffffff', #fff', 'ffffff', or 'fff'
myString.hexToRgb([array]);
"#123".hexToRgb(); //returns "rgb(17,34,51)" "112233".hexToRgb(); //returns "rgb(17,34,51)" "#112233".hexToRgb(true); //returns [17, 34, 51]
Converts an RGB color value to hexidecimal. Input string must be in one of the following RGB color formats. "rgb(255,255,255)", or "rgba(255,255,255,1)"
myString.rgbToHex([array]);
"rgb(17,34,51)".rgbToHex(); //returns "#112233" "rgb(17,34,51)".rgbToHex(true); //returns ['11','22','33'] "rgba(17,34,51,0)".rgbToHex(); //returns "transparent"
Strips the String of its <script>
tags and anything in between them.
myString.stripScripts([evaluate]);
var myString = "<script>alert('Hello')</script>Hello, World."; myString.stripScripts(); //Returns "Hello, World." myString.stripScripts(true); //Alerts "Hello", then returns "Hello, World."
Substitutes keywords in a string using an object/array. Removes undefined keywords and ignores escaped keywords.
myString.substitute(object[, regexp]);
var myString = "{subject} is {property_1} and {property_2}."; var myObject = {subject: 'Jack Bauer', property_1: 'our lord', property_2: 'savior'}; myString.substitute(myObject); //Jack Bauer is our lord and savior
© Linux.ria.ua, 2008-2024 |